Rose Garden Game | Mount Sinai Hospital
Description
The Rose Garden Game is a game developed in React.JS and JavaScript.
Code: https://github.com/emberlzhang/task_rose-garden_two-step
Purpose
The Rose Garden game was designed for the Center for Computational Psychiatry at Mount Sinai Hospital to differentiate between clinical populations with obsessive compulsive disorder (OCD) and healthy control (HC) populations. The task is inspired by the classic “two-stage” task paradigm in cognitive behavioral research.
Gameplay
The player plays rounds of two repeating choices followed in succession:
Choice 1: Pick a gardening store.
Choice 2: Pick a gardener.
After making these two choices, the player is rewarded with a rose planted in their rose garden. The rose is either yellow or red color, depending on the user choices made.
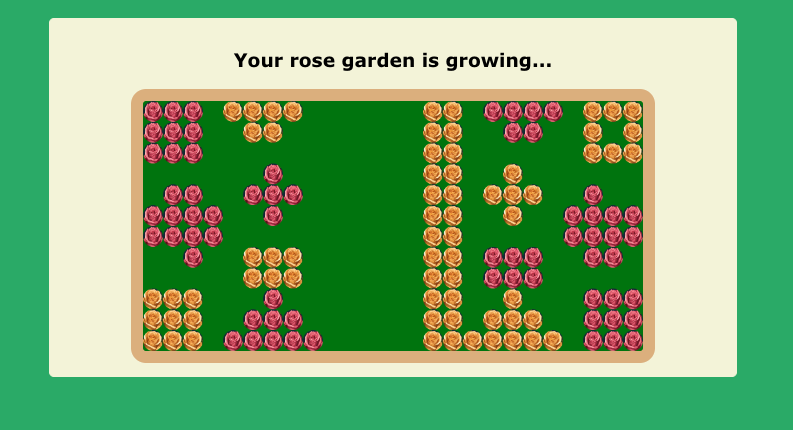
There are two gardening stores, and two pairs of gardeners in the game.
Over time, the player may come to notice, and make a preference for choosing flowers of all the same color, or choosing flowers of both colors, or some other such preference. This preference is of interest for researchers studying behavior compulsions, as it measures task perseverance.
Features
Probabilistic Game Logic
The choice of gardening store in Stage 1 and gardener in Stage 2 map probabilistically to picking red roses or yellow roses.
For example, in Stage 1, choosing a gardening store influences the selection of gardeners in Stage 2 based on probabilities. For instance, selecting a particular gardening store might give an 80% chance of being matched with one pair of gardeners and a 20% chance of being matched with a different pair.
Likewise, in Stage 2, the gardener chosen also influences the type of roses planted, again with probabilities. For example, selecting the gardener on the left side might result in an 80% chance of planting a red rose and a 20% chance of planting a yellow rose. Conversely, choosing the right-side gardener might give a 20% chance of planting a yellow rose and an 80% chance of planting a red rose.
Vector Based Rose Garden
A 2D array object is used to store the coordinates of roses within the rectangular garden, so that distinct “shapes” would appear, prompting the user to either explore further development of the shape with the same color rose, or explore alternatives using the other rose color. The rose “shape” configurations were generated by AI.
Try Ctrl+F “1” and “2” to see where the red and yellow roses populate, respectively.
// COLOR ASSIGNMENTS
// 1 = red rose
// 2 = yellow rose
export const flowerBeds = [
[
1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 1, 1,
],
[
1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 1, 1, 1,
],
[
0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 1, 1, 0,
],
[
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 2, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 0, 2, 2, 0, 0, 0, 1, 1, 0, 2, 2, 2, 2, 2, 2,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 1, 2, 2, 2, 2, 2, 0, 2, 0, 0, 2, 0, 0, 1, 0, 1, 1, 1, 1, 1, 2,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 1, 2, 1, 1, 2, 2, 0, 2, 0, 2, 2, 2, 0, 1, 0, 1, 1, 2, 2, 1, 2,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 1, 2, 1, 2, 2, 0, 0, 0, 0, 2, 2, 2, 0, 0, 0, 0, 1, 1, 2, 1, 2,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 1, 2, 2, 2, 0, 0, 0, 0, 2, 2, 1, 2, 2, 0, 0, 0, 0, 1, 1, 1, 2,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 1, 2, 2, 0, 0, 0, 2, 2, 2, 1, 1, 1, 2, 2, 2, 0, 0, 0, 1, 1, 2,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 2, 2, 1, 1, 1, 1, 1, 2, 2, 2, 0, 0, 0, 0, 0,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 2, 1, 1, 0, 0, 0, 2, 2, 2, 1, 1, 1, 2, 2, 2, 0, 0, 0, 2, 2, 1,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 2, 1, 1, 1, 0, 0, 0, 0, 2, 2, 1, 2, 2, 0, 0, 0, 0, 2, 2, 2, 1,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 2, 1, 2, 1, 1, 0, 0, 0, 0, 2, 2, 2, 0, 0, 0, 0, 2, 2, 1, 2, 1,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 2, 1, 2, 2, 1, 1, 0, 1, 0, 2, 2, 2, 0, 2, 0, 2, 2, 1, 1, 2, 1,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 2, 1, 1, 1, 1, 1, 0, 1, 0, 0, 2, 0, 0, 2, 0, 2, 2, 2, 2, 2, 1,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 2, 2, 2, 2, 2, 2, 0, 1, 1, 0, 0, 0, 2, 2, 0, 1, 1, 1, 1, 1, 1,
0, 0, 0, 0,
],
[
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 2, 2, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0,
],
[
0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 1, 1, 0,
],
[
1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 1, 1, 1,
],
[
1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 2, 2, 2, 2, 2, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 1, 1,
],
];
Selection Algorithm for Garden Rose Placement
Initially, a rose planting function would select a rose location at random based on the vector coordinate system. However, the “shapes” took too many rounds of game play before becoming apparent to the user. This made the feature less robust for the research study for which this task was intended.
Consequently, the development of a region-based rose selection algorithm allows the game to populate roses of one distinct “shape” region before moving to the next region, allowing the user to see the outcomes of their selection much earlier.
// Function to label regions for DFS
const labelRegions = (flowerBeds) => {
const regions = [];
const visited = flowerBeds.map((row) => row.map(() => false));
const dfs = (i, j, regionId) => {
if (i < 0 || j < 0 || i >= flowerBeds.length || j >= flowerBeds[0].length)
return;
if (flowerBeds[i][j] === 0 || visited[i][j]) return;
visited[i][j] = true;
regions[regionId].push([i, j]);
// Explore the four directions (up, down, left, right)
dfs(i - 1, j, regionId);
dfs(i + 1, j, regionId);
dfs(i, j - 1, regionId);
dfs(i, j + 1, regionId);
};
// Iterate over all flowerBeds to find regions
let regionId = 0;
for (let i = 0; i < flowerBeds.length; i++) {
for (let j = 0; j < flowerBeds[i].length; j++) {
if (flowerBeds[i][j] !== 0 && !visited[i][j]) {
regions[regionId] = [];
dfs(i, j, regionId);
regionId++;
}
}
}
return regions;
};
const regions = labelRegions(flowerBeds);
// Add flowers to a specific region
const addFlowersToRegion = (region, flowerType) => {
region.forEach(([i, j]) => {
flowerBeds[i][j] = flowerType; // Modify the flower bed with the flower type
});
};
// Prioritize adding flowers to one region at a time
let currentRegionIndex = 0;
const populateNextRegion = (flowerType) => {
if (currentRegionIndex < regions.length) {
addFlowersToRegion(regions[currentRegionIndex], flowerType);
currentRegionIndex++;
}
};
Significance to End User
The game tracks the player’s preference towards task perseveration vs. context switching, with the expectation of higher task perseveration for compulsive behavior populations relative to healthy control populations.
Player behavior and preferences are mapped to Bayesian inference models to discern group-level differences between HC populations and clinical behavioral compulsion groups.